The 3 basic types of design patterns you should know are creational, structural, and behavioral. Software design patterns help solve common problems in object-oriented design. Creational patterns like Singleton and Factory Method focus on object creation. Structural patterns, such as Adapter and Composite, deal with object composition. Behavioral patterns, including Observer and Strategy, manage object interactions. Choosing the right design pattern offers significant benefits and improves application efficiency.
3 Basic Types of Design Patterns You Should Know
When it comes to software development, design patterns are an integral part of the process. But what are design patterns? And what categories for design patterns exist? In this blog post, we’ll discuss the 3 basic types of design patterns you should be aware of: Creational, Structural, Behavioral, and Concurrency.
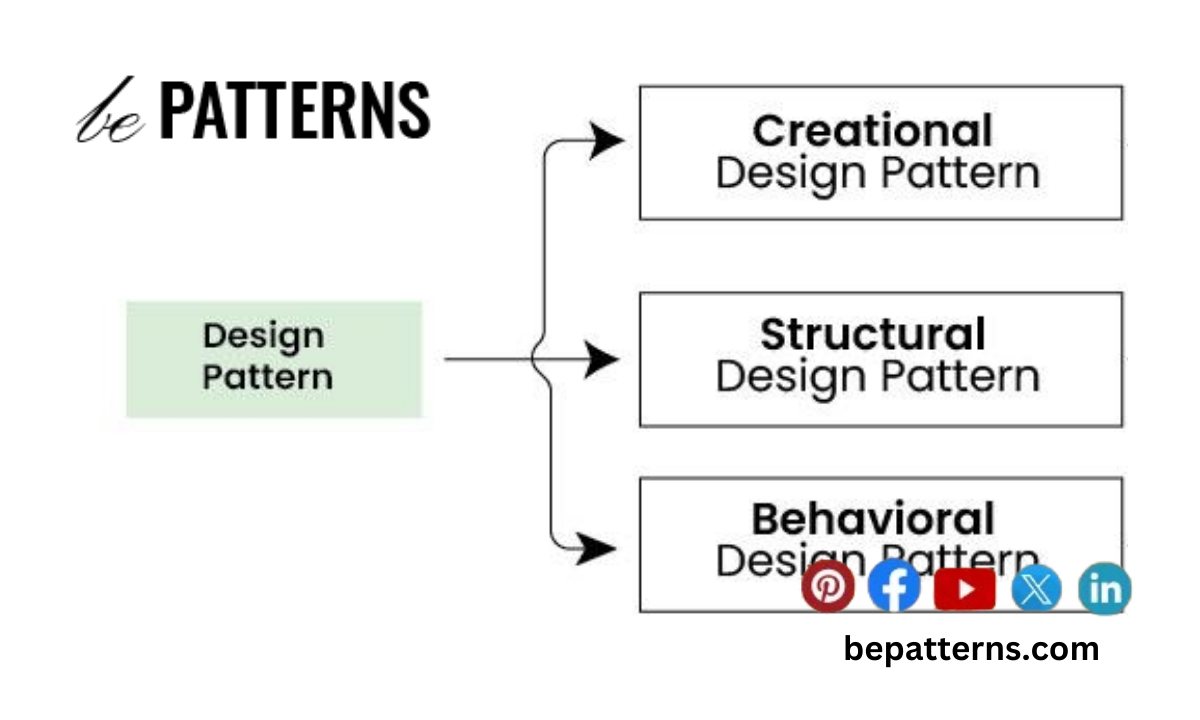
1. The Four Categories for Design Patterns
Design patterns are a powerful tool for solving common design and programming problems. They provide developers with the necessary tools to create efficient and maintainable software solutions. Generally, design patterns can be categorized into four major groups: creational, structural, behavioral, and concurrency.
The creational category:
The creational category of design patterns focuses on how an object is instantiated, composed, and represented. It includes patterns such as Factory Method, Abstract Factory, Builder, Prototype, Singleton, and more. These patterns are helpful for situations where there is a need to create objects consistently and to control the complexity of object creation.
The structural category:
The structural category of design patterns deals with the relationships between different types of objects and classes. It includes patterns such as Adapters, bridges, Composite, Decorators, Facades, Proxies, and more. These patterns provide ways to reduce the complexity of managing relationships between objects and classes.
The behavioral category:
The behavioral category of design patterns focuses on communication between objects. It includes patterns such as Chain of Responsibility, Command, Interpreter, Iterator, Mediator, Observer, State, Strategy, Template Method, and Visitor. These patterns help to identify common communication patterns between objects and consistently implement these interactions.
The concurrency category:
The concurrency category of design patterns addresses multi-threaded programming scenarios. It includes patterns such as Lock, Monitor Object, Thread Pool, Producer-Consumer, Barrier, Read Write Lock, and more. These patterns provide ways to handle concurrent programming tasks such as synchronization and thread management consistently.
2. The creational category
Design patterns in the creational category are concerned with how objects are created and initialized. The main goal of these design patterns is to provide an efficient way to create objects while reducing duplication of code and following object-oriented best practices.
The most commonly used design patterns in the creational category include Factory Method, Abstract Factory, Builder, Prototype, and Singleton. The Factory Method allows you to create a new instance of a class without knowing its concrete type, while the Abstract Factory is used to create related objects without having to specify their concrete classes. The Builder pattern helps to construct complex objects step by step, and the Prototype is used for creating a duplicate of an existing object. Lastly, the Singleton pattern is used to ensure that only one instance of a class exists at any given time.
3. The structural category
The structural category deals with how objects and classes can be combined to form larger structures. This could include items like creating objects that contain other objects, making sure that related objects are always kept in sync, or forming a class that acts as a wrapper for a group of related classes. These patterns are used to simplify the organization of code, create relationships between objects, and better handle complexity.
Some examples of structural design patterns include adapters, bridges, composite, decorators, flyweight, and proxy. The adapter pattern is used to link two incompatible components together. The bridge pattern allows different parts of a system to communicate without relying on each other. The composite pattern allows you to construct complex trees of objects and treat them in the same way.
The decorator pattern is used to wrap an object to add additional functionality to it. The flyweight pattern enables objects to share data to reduce the overhead of creating and storing multiple copies of the same object. Finally, the proxy pattern acts as an intermediary between two components, allowing them to interact without directly exposing one another.