When considering Python design patterns, it’s essential to understand which one suits your project. Python offers various design patterns, including creational, structural, and behavioral patterns. For instance, the singleton pattern ensures only one instance of a class exists, while the factory pattern creates objects without specifying their exact class.
Depending on your project’s requirements, choosing the right design pattern can enhance code readability and maintainability. Explore examples and tutorials to grasp each pattern’s usage effectively.
Python design patterns which one should you use?
Python design patterns which one should you use? Are you wondering which design pattern is used in Python? Choosing the right design pattern for a project can be daunting, especially if you’re new to the programming language. This blog post will provide an overview of the most commonly used Python design patterns and explain how each one can help you build better applications. We’ll explore the advantages and disadvantages of each one so you can make an informed decision about which design pattern is best for your project.
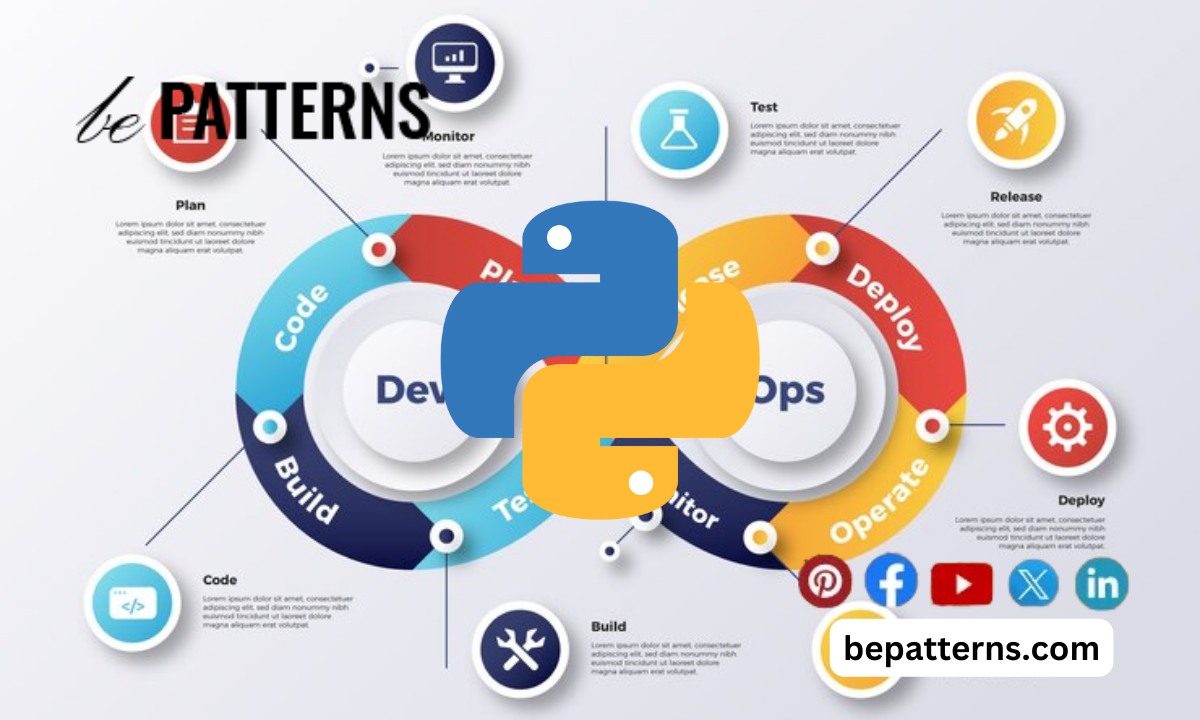
1. Factory Method
The Factory Method design pattern is one of the most widely used patterns in Python programming. It’s a creational pattern that uses factory methods to create objects without having to specify their class type. The goal of this pattern is to create objects in a way that’s easier to manage and maintain.
In Python, the Factory Method is defined using a method called “factory”. This method takes an argument which is typically a class type or the name of a class. The factory method then instantiates an object of that class type and returns it. This allows you to use objects without knowing their class types and makes your code more flexible.
For example, if you have an application that needs to generate a random number, you could use the Factory Method to create an object that can generate random numbers of any kind. You wouldn’t have to explicitly define the class type of the object you need, as the Factory Method would take care of this for you.
The Factory Method is a great way to make your code more flexible and maintainable. It also makes it easier to extend your code by adding new classes without breaking existing code.
2. Singleton
The Singleton design pattern is a useful tool when you want to make sure that only one instance of a class is created and accessible. This is particularly helpful when a particular object needs to be accessed by multiple components or applications within a program.
In Python, the Singleton design pattern can be implemented in several ways. One way is to make use of the singleton module, which provides a base class that can be used to create singleton objects. This class is designed so that only one object is ever instantiated from the class, and any other attempts to create additional instances of the same class will return the existing instance instead.
To create a singleton object in Python, simply inherit from the singleton base class and ensure that the class has an init method with no parameters. From there, you can add additional methods and attributes as needed to complete your singleton object. Once the object is created, it can be accessed from any point in your code.
When considering whether to implement a singleton in Python, it’s important to remember that singletons are generally only recommended for specific situations. As such, make sure to evaluate your particular problem carefully before deciding to use the Singleton design pattern.
3. Observer
The Observer pattern is a popular design pattern used in software development. It is also commonly referred to as the publish-subscribe pattern and is used to allow for one-to-many communication between objects or components. In this design pattern, the ‘subject’, which is typically an object or component, will keep track of any observers that may be interested in receiving updates from it. Whenever the subject makes a change, it will notify all the observers who are subscribed to it so they can take the appropriate action.
The Observer pattern is beneficial because it helps to promote loose coupling between objects or components. This means that changes in one area of the code won’t affect the others. It also simplifies making updates by allowing you to notify all of your observers at once, instead of having to update each one individually.
In Python, the Observer pattern is usually implemented using the built-in observer library. The library provides classes for defining subjects, observers, and events. It also provides an interface for observers to register themselves with the subject. When the subject updates its state, the library notifies all of the registered observers and allows them to take action.
The Observer pattern can be used in many different applications including GUI development, event-driven programming, distributed systems, and even in-game development. By leveraging the Observer pattern, you can ensure that your codebase is maintainable and that changes won’t affect other areas of your code. If you’re looking for a way to efficiently implement one-to-many communication in Python, then the Observer pattern is worth considering.