Structural design patterns are solutions to common problems in software architecture, focusing on how classes and objects are composed to form larger structures. These patterns, like those in Java, enhance code reusability and maintainability. While structural design patterns differ from creational design patterns, both are part of the Gang of Four design principles, crucial for managing software complexity and scalability in object-oriented programming.
What are Structural Design Patterns?
Structural design patterns help simplify the design of software by ensuring that different parts work together seamlessly. Examples include the Adapter pattern, which allows incompatible interfaces to communicate, and the Facade pattern, which provides a simplified interface to complex systems. Unlike creational design patterns, which focus on object creation, structural patterns deal with object composition and relationships. In C#, these patterns include Composite, Decorator, and Proxy.
Structural design patterns are an important part of software development, but what exactly are they? In this blog post, we’ll take a look at what structural design patterns are, their various types, and how they can be used to improve the architecture and design of your applications. We’ll also go over the advantages and disadvantages of each type of pattern and some best practices for implementation. By the end of this post, you’ll have a better understanding of structural design patterns and how to apply them to your projects.

Definition of a Structural Design Pattern
A structural design pattern is a type of software design pattern that focuses on the relationship and organization between objects. This type of pattern is used to create a more efficient, logical, and reusable structure for an application’s code. It can help developers better organize their code by defining a consistent way of connecting classes or objects within an application. Structural design patterns use inheritance to compose interfaces and classes into larger structures, allowing developers to reuse existing components instead of building custom solutions.
Structural design patterns are categorized into seven main types: Adapter, Bridge, Composite, Decorator, Facade, Flyweight, and Proxy. Each one provides its own set of benefits and can be used in different scenarios. They help developers quickly identify, create, and maintain relationships between objects while ensuring code readability and maintainability.
The 7 Most Popular Structural Design Patterns
1. Adapter Pattern:
The Adapter Pattern is a structural design pattern that allows objects with different interfaces to work together by converting the interface of one object into another. It can be used when two classes have incompatible interfaces, or when one class wants to reuse an existing class with a different interface.
2. Bridge Pattern:
The Bridge Pattern is a structural design pattern that allows a class to be implemented as two separate parts, each part separately varying. This pattern is typically used in situations where a subclass needs to vary from the parent class, but both classes must remain compatible.
3. Composite Pattern:
The Composite Pattern is a structural design pattern that lets you treat a group of objects similar to how you would treat a single object. It can be used to create hierarchical structures and can also help reduce complexity.
4. Decorator Pattern:
The Decorator Pattern is a structural design pattern that lets you dynamically add features to an object without changing the object’s code. It can be used to enhance the behavior of an object by wrapping it in an object of a decorator class.
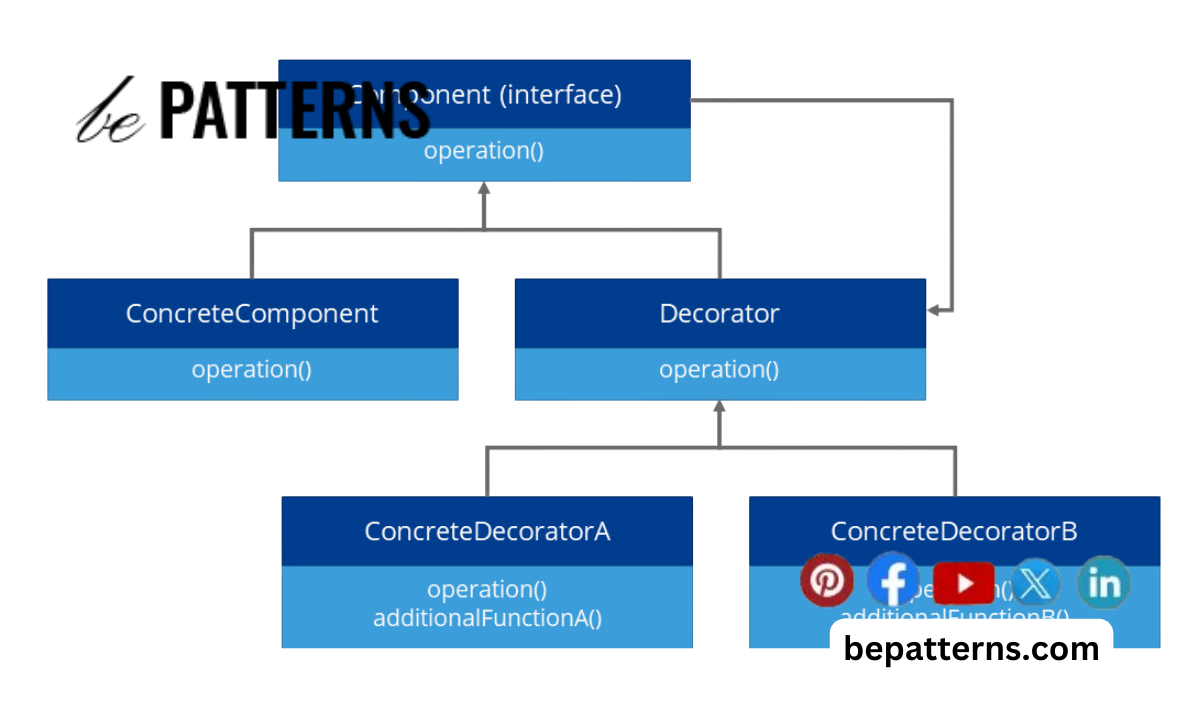
5. Facade Pattern:
The Facade Pattern is a structural design pattern that provides an interface to access a complex system. It can be used to simplify complex system interactions and improve usability by providing a single point of access for many services and operations.
6. Flyweight Pattern:
The Flyweight Pattern is a structural design pattern that helps reduce memory consumption by sharing common data between objects. It can be used when a large number of objects with similar characteristics exist, allowing them to share information and reduce the overall amount of memory used.
7. Proxy Pattern:
The Proxy Pattern is a structural design pattern that provides an interface to access an object indirectly. It can be used to control access to an object, hiding its complexities and protecting it from unnecessary access.